Getting information from the QPU
QPUs define a set of specifications, stored in an object of type HardwareSpecs
, which can be retrieved by get_specs()
. This set of specifications defines
what could be executed on the QPU, for instance:
the number of qubits composing the QPU
the topology of the QPU (i.e. pair of qubits on which a two-qubit gate can be applied - this attribute is a graph of type
Topology
)the gateset of the QPU (i.e. supported gates)
the processing types (i.e. if the QPU support SAMPLE measurement, OBSERVABLE measurement, or both)
the description of the QPU
any meta_data that the QPU provides to the user, which can be any custom data, represented in a dictionary that maps strings to strings
These specifications can be used by plugins to adapt their compilation stage, to target the QPU
Creating custom specifications
This section provides an example explaining how to create custom hardware specs. In this example, we will consider a QPU capable of executing RX, RZ and CNOT gates. CNOT gates can only be applied between a few pairs of qubits defined by the topology below. This QPU is assumed to support SAMPLE measurement
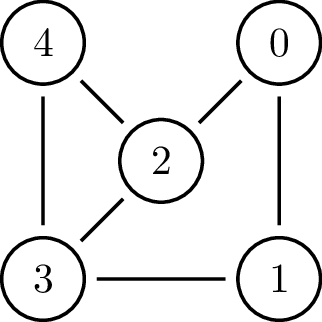
This topology can be created using networkx and cast into a Topology
:
import networkx as nx
from qat.core import Topology
graph = nx.Graph([(0, 1), (0, 2), (1, 3), (2, 3), (2, 4), (3, 4)])
topology = Topology.from_nx(graph)
The gate-set can be defined using gates defined in qat.lang
:
from qat.core.gate_set import GateSet
from qat.lang import RX, RZ, CNOT
gateset = GateSet({"RX": RX, "RZ": RZ, "CNOT": CNOT})
Now, hardware specifications can be merged into a single object:
from qat.core import HardwareSpecs
from qat.comm.shared.ttypes import ProcessingType
my_specs = HardwareSpecs(nbqbits=5, topology=topology, gateset=gateset, processing_types=[ProcessingType.SAMPLE],
description="Example of QPU specs", meta_data={ "last calibration date": "2024-03-18 15:50:00" })